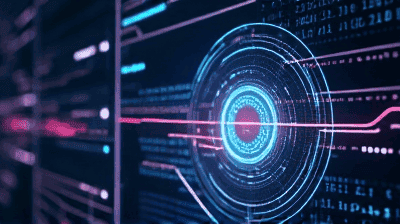
In recent years, quantum computing has emerged as a groundbreaking field that promises to revolutionize the way we process information. Unlike classical computers that operate using bits as the smallest unit of data, quantum computers utilize qubits, which leverage the principles of quantum mechanics. But what does it mean to program in this new realm of qubits? How do we harness the power of quantum mechanics to write effective code?
Understanding Classical Bits and Quantum Qubits
What is a Bit?
A bit is the fundamental unit of information in classical computing. It can exist in one of two states: 0 or 1. Classical computers manipulate these bits using logical operations to perform computations, from basic arithmetic to complex algorithms.
Introducing Qubits
Qubits, or quantum bits, are the fundamental units of quantum information. They differ significantly from classical bits due to the principles of superposition and entanglement. A qubit can exist in a state of 0, 1, or any quantum superposition of both states, allowing for much richer representations of information.
Superposition: While a classical bit can only be in one state at a time, a qubit can simultaneously represent both 0 and 1 until it is measured. This feature provides quantum computers with the ability to process vast amounts of data in parallel, leading to a potential exponential increase in computational power.
Entanglement: Qubits can become entangled, a phenomenon where the state of one qubit is directly related to the state of another, no matter the distance between them. Entangled qubits allow complex correlations between particles, contributing to the quantum computer's ability to solve problems that are currently intractable for classical computers.
The Principles of Quantum Computing
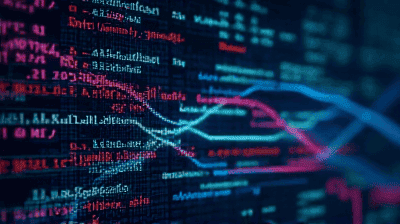
Quantum Gates
In quantum programming, quantum gates serve as the fundamental building blocks, analogous to classical logic gates. Quantum gates manipulate qubits through unitary operations, transforming their states according to the rules of quantum mechanics. Examples of quantum gates include:
- Hadamard Gate (H): Creates superposition by transforming a qubit into an equal probability of being measured as 0 or 1.
- Pauli-X Gate: Functions like a classical NOT gate, flipping the state of a qubit.
- CNOT Gate (Controlled-NOT): A two-qubit gate that flips the state of the target qubit if the control qubit is in the state 1.
Quantum Circuits
Quantum circuits are the framework used to design quantum algorithms. A quantum circuit consists of a series of quantum gates applied to a set of qubits. The output of these circuits can be measured to obtain classical results, allowing for the execution of algorithms on quantum hardware. Designing efficient quantum circuits is crucial for optimizing the performance of quantum algorithms.
Measurement in Quantum Computing
Measurement collapses the state of a qubit from a superposition to a definitive classical state (either 0 or 1). The probabilistic nature of quantum measurement means that running the same quantum circuit multiple times may yield different results, providing a measure of the likelihood of each outcome based on the quantum state prior to measurement.
Quantum Programming Languages
Programming quantum computers requires specialized languages designed to handle qubits and quantum operations. Several quantum programming languages have been developed to facilitate quantum algorithm implementation:
Qiskit
Developed by IBM, Qiskit is an open-source quantum computing framework that provides tools for creating quantum programs using Python. Qiskit allows developers to design quantum circuits, run simulations, and execute programs on real quantum hardware.
- Key Features:
- Quantum circuit creation and visualization
- Integration with IBM Quantum Experience for access to real quantum devices
- Powerful tools for quantum algorithm development
Cirq
Cirq is a Python library developed by Google for building and simulating quantum circuits. It is particularly well-suited for designing quantum algorithms that can be executed on Google’s quantum processors.
- Key Features:
- Tools for creating custom quantum gates
- Support for noise modeling and error mitigation
- Easy integration with reinforcement learning frameworks
Q# and the Quantum Development Kit (QDK)
Q# is a programming language developed by Microsoft specifically for quantum programming. The Quantum Development Kit encompasses various tools and libraries to aid developers in creating quantum algorithms.
- Key Features:
- Strong integration with classical programming languages
- A rich library of quantum algorithms and operations
- Support for quantum simulations and debugging
Writing Your First Quantum Program
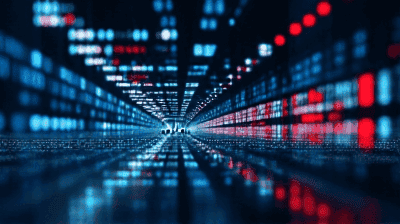
To give you a practical sense of how quantum programming works, let’s walk through a simple example using Qiskit. We will create a quantum circuit that demonstrates the creation of a superposition state using the Hadamard gate.
Step 1: Setting Up Your Environment
Before you begin, make sure you have Python and Qiskit installed. You can easily install Qiskit using pip:
bash 1pip install qiskit 2
Step 2: Importing Qiskit
Start by importing the necessary components from Qiskit:
python 1from qiskit import QuantumCircuit, Aer, transpile, execute 2from qiskit.visualization import plot_histogram 3
Step 3: Creating a Quantum Circuit
We will create a quantum circuit with one qubit and apply the Hadamard gate:
python 1# Create a Quantum Circuit with 1 qubit 2qc = QuantumCircuit(1) 3 4# Apply the Hadamard gate 5qc.h(0) 6 7# Measure the qubit to collapse its state 8qc.measure_all() 9
Step 4: Executing the Circuit
Now, we can execute the circuit using a simulator:
python 1# Use the AER simulator 2simulator = Aer.get_backend('aer_simulator') 3 4# Transpile the circuit for the simulator 5transpiled_circuit = transpile(qc, simulator) 6 7# Execute the circuit 8job = execute(transpiled_circuit, simulator, shots=1000) 9 10# Get the results 11results = job.result() 12counts = results.get_counts() 13 14# Plot the results 15plot_histogram(counts) 16
Step 5: Understanding the Output
When you execute the above script, you should see a histogram showing the probability distribution of measuring 0 and 1. Due to the Hadamard gate, the qubit is in a superposition state, which means you should observe approximately equal counts for both outcomes across the 1000 shots.
Quantum Algorithms
Grover's Algorithm
Grover's algorithm is a well-known quantum search algorithm that provides a quadratic speedup for unstructured search problems. Instead of linearly searching through an unsorted database, Grover’s algorithm allows you to find the target item in approximately the square root of the total number of items.
Key Steps in Grover's Algorithm:
- Initialization: Prepare qubits in a superposition of all possible states.
- Oracle: Use an oracle function that marks the target state by inverting its amplitude.
- Amplification: Apply Grover’s diffusion operator to amplify the probability of the target state.
- Measurement: Measure the qubits to retrieve the target item.
Shor's Algorithm
Shor's algorithm revolutionizes number factorization and provides exponential speedup compared to the best-known classical algorithms. It is especially significant in the context of cryptography, where breaking widely-used encryption methods hinges on factorization.
Key Steps in Shor's Algorithm:
- Choose a random number and find a period of the function, which reduces the problem to finding the greatest common divisor.
- Quantum Fourier Transform: Use the quantum Fourier transform to extract the period.
- Measure: Utilize classical post-processing to find the factors of the original number.
Challenges in Quantum Programming
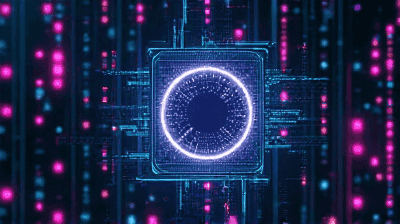
While the potential of quantum programming is enormous, several challenges must be addressed to fully realize its capabilities:
1. Noise and Decoherence
Quantum computers are highly susceptible to noise and errors due to their reliance on fragile quantum states. Decoherence can cause the loss of information and lead to inaccurate computations. Researchers are actively exploring error correction techniques and fault-tolerant quantum computing to mitigate these issues.
2. Scalability
Building scalable quantum systems remains a significant challenge. Current quantum processors have a limited number of qubits, which constrains the complexity of the algorithms that can be executed. Innovations in qubit architecture and interconnectivity are crucial for scaling quantum computers.
3. Developing Quantum Algorithms
There remains a limited number of known quantum algorithms compared to classical counterparts. While some problems show promise for quantum speedup, finding and developing new algorithms that can benefit from quantum computing is an ongoing area of research.
The Future of Quantum Programming
As quantum computing technology matures, the demand for quantum programmers will increase. Understanding the principles of quantum mechanics, quantum circuits, and programming languages will be essential for professionals in various fields, including computer science, finance, cryptography, and materials science.
Education and Resources
To thrive in quantum programming, consider engaging with the following resources:
- Online Courses: Platforms like Coursera and edX offer courses on quantum computing and programming.
- Books: Titles such as "Quantum Computation and Quantum Information" by Michael Nielsen and Isaac Chuang provide in-depth knowledge on the subject.
- Community Engagement: Join online forums and communities, such as the Quantum Computing Stack Exchange or Qiskit’s community, to collaborate and learn from others.
Conclusion
Quantum programming represents an exciting frontier in computer science, harnessing the power of qubits to solve complex problems far beyond the reach of classical computation. While there are still challenges to overcome, the foundational concepts of superposition, entanglement, and quantum algorithms offer a glimpse into a new era of computing.
With ongoing advancements in quantum hardware and programming languages, the barriers to entry are gradually lowering, making it an opportune time for aspiring quantum programmers to dive into the field. By acquiring knowledge and skills in quantum programming, you can become part of a transformative movement that will shape the future of technology and computation.